Introduction
In this comprehensive tutorial, we will create a media player with a frequency-based visual equalizer using JavaScript, CSS, and HTML. By leveraging the Web Audio API, we can analyze the audio frequencies in real-time and provide a dynamic visualization that enhances the user experience. This project will help you understand how to integrate audio processing and visualization in a web application.
Full Code Snippet
Here is the complete code for the media player with a frequency-based visual equalizer:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Frequency-based Media Player with Visual Equalizer</title>
<style>
/* Basic styling for body */
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #282c34;
color: white;
font-family: Arial, sans-serif;
}
/* Styling for the audio player */
#audio-player {
margin-bottom: 20px;
}
/* Flex container for equalizer bars */
.equalizer {
display: flex;
gap: 5px;
}
/* Individual bar styling */
.bar {
width: 10px;
background: linear-gradient(to top, #ff0000, #ffff00, #00ff00);
transition: height 0.1s; /* Smooth transition for height changes */
}
</style>
</head>
<body>
<h1>Media Player with Visual Equalizer</h1>
<audio id="audio-player" controls>
<source src="your-audio-file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
<div class="equalizer" id="equalizer">
<!-- Equalizer bars -->
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
</div>
<script>
// Get the audio player and equalizer elements
const audioPlayer = document.getElementById('audio-player');
const equalizer = document.getElementById('equalizer');
const bars = equalizer.getElementsByClassName('bar');
// Create audio context and analyzer
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
const analyzer = audioContext.createAnalyser();
analyzer.fftSize = 256; // Fast Fourier Transform size
const bufferLength = analyzer.frequencyBinCount;
const dataArray = new Uint8Array(bufferLength);
// Connect the audio element to the analyzer
const source = audioContext.createMediaElementSource(audioPlayer);
source.connect(analyzer);
analyzer.connect(audioContext.destination);
// Function to render the frequency data
function renderFrame() {
requestAnimationFrame(renderFrame); // Call renderFrame recursively
analyzer.getByteFrequencyData(dataArray); // Get frequency data
// Update bar heights based on frequency data
for (let i = 0; i < bars.length; i++) {
const barHeight = dataArray[i];
bars[i].style.height = `${barHeight}px`;
}
}
// Event listener to start the visualization when audio plays
audioPlayer.addEventListener('play', () => {
audioContext.resume().then(() => {
renderFrame(); // Start rendering frames
});
});
</script>
</body>
</html>
Explanation
HTML Structure
The HTML structure includes the audio element and the visual equalizer. The audio
element allows users to play and control the audio file. The equalizer is represented by a series of div
elements, each with the class bar
.
<audio id="audio-player" controls>
<source src="your-audio-file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
<div class="equalizer" id="equalizer">
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
<div class="bar" style="height: 10px;"></div>
</div>
CSS Styling
The CSS styles the media player and equalizer. Each bar
element is animated based on the frequency data. We have used a linear gradient for the bars and smooth transitions for height changes to make the visual effect more appealing.
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #282c34;
color: white;
font-family: Arial, sans-serif;
}
#audio-player {
margin-bottom: 20px;
}
.equalizer {
display: flex;
gap: 5px;
}
.bar {
width: 10px;
background: linear-gradient(to top, #ff0000, #ffff00, #00ff00);
transition: height 0.1s;
}
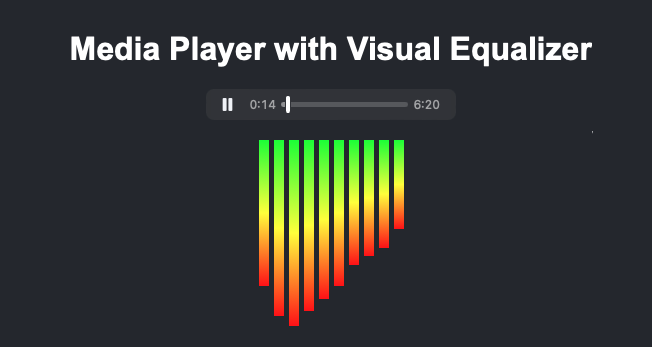
JavaScript Functionality
The JavaScript code handles the interaction between the audio player and the visual equalizer using the Web Audio API. Here is a step-by-step explanation:
- Create Audio Context and Analyzer:
First, we create an audio context and an analyzer. The analyzer will allow us to get frequency data from the audio.
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
const analyzer = audioContext.createAnalyser();
analyzer.fftSize = 256; // Fast Fourier Transform size
const bufferLength = analyzer.frequencyBinCount;
const dataArray = new Uint8Array(bufferLength);
- Connect Audio Element to Analyzer:
Next, we connect the audio element to the analyzer. This step is crucial as it allows the analyzer to process the audio data.
const source = audioContext.createMediaElementSource(audioPlayer);
source.connect(analyzer);
analyzer.connect(audioContext.destination);
- Render Frame Function:
The renderFrame
function updates the height of each bar based on the frequency data. It uses requestAnimationFrame
to ensure smooth animation.
function renderFrame() {
requestAnimationFrame(renderFrame); // Call renderFrame recursively
analyzer.getByteFrequencyData(dataArray); // Get frequency data
// Update bar heights based on frequency data
for (let i = 0; i < bars.length; i++) {
const barHeight = dataArray[i];
bars[i].style.height = `${barHeight}px`;
}
}
- Play Event Listener:
We add an event listener to the audio player that starts the visualization when the audio plays.
audioPlayer.addEventListener('play', () => {
audioContext.resume().then(() => {
renderFrame(); // Start rendering frames
});
});
Additional Customizations
You can further customize the media player and equalizer by making a few changes to the code. Here are some options:
- Change the Number of Bars:
To change the number of bars, add or remove div
elements with the class bar
inside the equalizer
div in the HTML. Adjust the fftSize
property in the JavaScript accordingly.
<div class="equalizer" id="equalizer">
<!-- Add more bars for a finer visualization -->
<div class="bar" style="height: 10px;"></div>
<!-- Repeat for more bars -->
</div>
- Modify Bar Colors:
Change the colors of the bars by modifying the background
property in the CSS. Use different color values or gradients to achieve the desired effect.
.bar {
width: 10px;
background: linear-gradient(to top, #0000ff, #00ffff, #00ff00);
transition: height 0.1s;
}
- Adjust Animation Speed:
Modify the transition
property in the CSS to change the speed of the height transitions.
.bar {
width: 10px;
background:
linear-gradient(to top, #ff0000, #ffff00, #00ff00);
transition: height 0.05s; /* Faster transition */
}
- Autoplay Audio:
To autoplay the audio when the page loads, add the autoplay
attribute to the audio
tag.
<audio id="audio-player" controls autoplay>
<source src="your-audio-file.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
- Add Volume Control:
To add volume control, you can include a range input element and adjust the audio volume dynamically.
<input type="range" id="volume-control" min="0" max="1" step="0.01" value="1">
const volumeControl = document.getElementById('volume-control');
volumeControl.addEventListener('input', (event) => {
audioPlayer.volume = event.target.value;
});
Practical Usage
This frequency-based media player can be embedded in any website to provide audio content with dynamic visualization. The equalizer enhances the user experience by visualizing the audio frequencies in real-time. You can customize the number of bars and their appearance to fit your website’s design. Experimenting with different styles and functionalities will help you create a unique and engaging media player.
Questions and Answers
Q: How can I change the colors of the equalizer bars?
A: You can change the colors by modifying the background
property of the .bar
class in the CSS. Use different color values or gradients to achieve the desired effect.
Q: How can I increase the number of equalizer bars?
A: To increase the number of bars, add more div
elements with the class bar
inside the equalizer
div in the HTML. Ensure the fftSize
in the JavaScript is adjusted accordingly.
Q: Can I use different audio formats with this media player?
A: Yes, you can use different audio formats by adding multiple source
elements within the audio
tag, specifying different formats like MP3, OGG, or WAV.
Q: How can I make the equalizer more responsive?
A: To make the equalizer more responsive, adjust the fftSize
property of the analyzer. Higher values provide more frequency data, making the visualization smoother.
Q: How can I autoplay the audio when the page loads?
A: To autoplay the audio, add the autoplay
attribute to the audio
tag: <audio id="audio-player" controls autoplay>
.
Related Subjects
Web Audio API
The Web Audio API allows for complex audio processing and manipulation in web applications. It can be used to create more advanced visualizations that respond to audio frequencies in real-time. Learn more about the Web Audio API on the MDN Web Docs.
CSS Animations
CSS animations are used to create visual effects and dynamic content on web pages. Understanding CSS animations can enhance your ability to create engaging user interfaces. Explore CSS animations on the W3Schools website.
JavaScript Event Listeners
Event listeners in JavaScript allow you to execute code in response to user actions, such as playing or pausing audio. Mastering event listeners is crucial for creating interactive web applications. Check out more about event listeners on the JavaScript.info website.
HTML5 Audio Element
The HTML5 audio element is used to embed audio content in web pages. It provides a simple way to add media playback functionality. Learn more about the HTML5 audio element on the HTML5 Doctor website.
Conclusion
In this article, we have demonstrated how to create a media player with a frequency-based visual equalizer using JavaScript, CSS, and HTML. By following the steps and understanding the code provided, you can build and customize your own media player. Experiment with different styles and functionalities to enhance your web projects. If you have any questions or need further assistance, feel free to ask in the comments.