Introduction
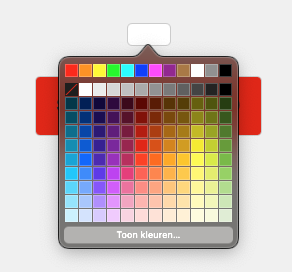
A color picker is a handy tool that allows users to select a color from a palette. It’s widely used in web applications, particularly those involving design or customization features. In this article, we will demonstrate how to create a simple yet functional color picker using JavaScript, HTML, and CSS.
Setting Up the Color Picker
To build our color picker, we will use HTML for the structure, CSS for styling, and JavaScript for functionality. By following these steps, you’ll have a fully functional color picker ready for use in your projects.
Code Snippet and Explanation
HTML Structure
First, let’s set up the basic HTML structure. We’ll create an input field of type color and a div to display the selected color.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Color Picker</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="color-picker-container">
<input type="color" id="color-picker">
<div id="color-display">Selected Color</div>
</div>
<script src="script.js"></script>
</body>
</html>
In this HTML snippet, we include a color-picker
input and a color-display
div to show the chosen color. We also link to an external CSS file (styles.css
) and a JavaScript file (script.js
).
CSS Styling
Next, we’ll add some basic styles to make our color picker look nice.
/* styles.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
.color-picker-container {
text-align: center;
}
#color-picker {
margin: 20px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
#color-display {
margin-top: 10px;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
color: #333;
font-weight: bold;
}
This CSS code centers the color picker on the page and styles both the input field and the display div for better aesthetics.
JavaScript Functionality
Finally, let’s implement the JavaScript to handle color selection and display.
// script.js
document.addEventListener('DOMContentLoaded', function() {
const colorPicker = document.getElementById('color-picker');
const colorDisplay = document.getElementById('color-display');
colorPicker.addEventListener('input', function() {
const selectedColor = colorPicker.value;
colorDisplay.style.backgroundColor = selectedColor;
colorDisplay.textContent = `Selected Color: ${selectedColor}`;
});
});
This JavaScript code listens for changes on the color input and updates the background color and text of the color-display
div accordingly.
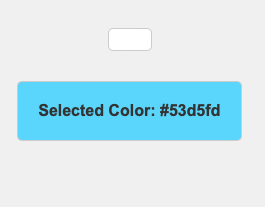
Practical Usage
This color picker can be used in various scenarios, such as:
- Allowing users to customize the colors of UI elements in a web application.
- Providing a way to pick colors for designing graphics or themes.
- Integrating into a form where users need to choose their favorite color.
Example and Comparison
Compared to third-party libraries like Spectrum or Pickr, our custom color picker is lightweight and easy to integrate. However, third-party libraries offer more advanced features like color palettes, presets, and more customizable options.
Related Subjects:
- Creating Custom Color Palettes in CSS: Learn how to define and use custom color palettes for consistent styling across your website. Mozilla Developer Network (MDN)
- Using JavaScript to Manipulate CSS: Understand how to dynamically change CSS styles using JavaScript for interactive web pages. W3Schools
- Advanced Color Pickers: Explore advanced color picker libraries like Pickr for more features and customization options. Pickr Library
- HTML5 Input Types: Discover the variety of input types available in HTML5, including color input. HTML5 Doctor
Conclusion
Creating a color picker with JavaScript, HTML, and CSS is a straightforward task that adds valuable functionality to web applications. By following this guide, you can implement a simple color picker and customize it to fit your needs. If you have any questions or suggestions, feel free to leave a comment below. Try integrating this color picker into your next project today!