Create a Character Counter with JavaScript, HTML, and CSS
Introduction
In this blog, we will learn how to create a character counter using JavaScript, HTML, and CSS. A character counter is a handy tool often used in text input fields to show users the number of characters they have typed. This feature is particularly useful for applications like social media platforms or text editors where character limits are enforced. We will walk through the process of building this feature step-by-step, ensuring you understand how each part works.
Languages and Techniques
To build this character counter, we will use:
- HTML for structuring the content.
- CSS for styling the appearance.
- JavaScript for the functionality.
Full Code Snippet
Below is the complete code for our character counter. The HTML provides the structure, CSS handles the styling, and JavaScript implements the logic. Additionally, we’ve included comments to explain each part of the code for better understanding.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Character Counter</title>
<style>
/* Basic styling for the body */
body {
font-family: Arial, sans-serif;
margin: 50px;
}
/* Container for the textarea and counter */
.counter-container {
display: flex;
flex-direction: column;
max-width: 300px;
}
/* Styling for the textarea */
textarea {
resize: none; /* Prevents resizing */
width: 100%;
height: 100px;
margin-bottom: 10px;
padding: 10px; /* Add padding for better appearance */
border: 1px solid #ccc; /* Light gray border */
border-radius: 5px; /* Rounded corners */
font-size: 16px; /* Slightly larger font size */
}
/* Default styling for the character counter */
.counter {
font-size: 14px;
color: #333;
}
/* Warning style for the counter when nearing the character limit */
.warning {
color: red;
}
</style>
</head>
<body>
<!-- Container holding the textarea and the character counter -->
<div class="counter-container">
<textarea id="textInput" maxlength="150" placeholder="Type something..."></textarea>
<div class="counter">Characters: <span id="charCount">0</span></div>
</div>
<script>
// Get references to the textarea and counter elements
const textInput = document.getElementById('textInput');
const charCount = document.getElementById('charCount');
// Maximum length for the input
const maxLength = 150;
// Threshold to trigger the warning style
const warningThreshold = 100;
// Flag to show remaining characters instead of the count
const showRemaining = true;
// Add event listener to update the character count on input
textInput.addEventListener('input', () => {
// Get the current length of the text input
const length = textInput.value.length;
// Update the character count or remaining characters
if (showRemaining) {
charCount.textContent = maxLength - length;
} else {
charCount.textContent = length;
}
// Apply warning style if the threshold is reached
if (length > warningThreshold) {
charCount.classList.add('warning');
} else {
charCount.classList.remove('warning');
}
// Display custom message when the limit is reached
if (length >= maxLength) {
charCount.textContent = "You have reached the limit!";
} else if (length > warningThreshold) {
charCount.textContent = `Warning: Only ${maxLength - length} characters left`;
} else {
charCount.textContent = `Characters: ${length}`;
}
});
</script>
</body>
</html>
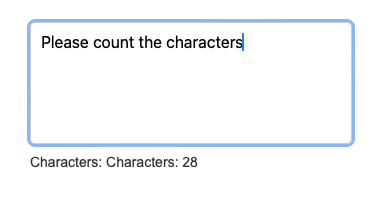
Step-by-Step Explanation
HTML Structure
The HTML part is quite simple. It includes a textarea
for text input and a div
to display the character count. The textarea
has an id
of textInput
, which we will use to reference it in our JavaScript code. The character counter is displayed inside a div
with the class counter
, and the actual count is inside a span
with the id
charCount
.
<div class="counter-container">
<textarea id="textInput" maxlength="150" placeholder="Type something..."></textarea>
<div class="counter">Characters: <span id="charCount">0</span></div>
</div>
CSS Styling
In the CSS section, we style the body, textarea, and counter. We use Flexbox to organize the elements within the container. The textarea is made non-resizable to maintain the layout, and a warning style is defined to change the counter’s color when a certain threshold is reached.
/* Basic styling for the body */
body {
font-family: Arial, sans-serif;
margin: 50px;
}
/* Container for the textarea and counter */
.counter-container {
display: flex;
flex-direction: column;
max-width: 300px;
}
/* Styling for the textarea */
textarea {
resize: none; /* Prevents resizing */
width: 100%;
height: 100px;
margin-bottom: 10px;
padding: 10px; /* Add padding for better appearance */
border: 1px solid #ccc; /* Light gray border */
border-radius: 5px; /* Rounded corners */
font-size: 16px; /* Slightly larger font size */
}
/* Default styling for the character counter */
.counter {
font-size: 14px;
color: #333;
}
/* Warning style for the counter when nearing the character limit */
.warning {
color: red;
}
JavaScript Functionality
The JavaScript part adds an event listener to the textarea
. Whenever the user types, the script updates the character count. The character count can be customized to show either the number of characters typed or the number of characters remaining. Additionally, a warning style is applied when the character count exceeds a predefined threshold, and custom messages are displayed when the limit is reached.
// Get references to the textarea and counter elements
const textInput = document.getElementById('textInput');
const charCount = document.getElementById('charCount');
// Maximum length for the input
const maxLength = 150;
// Threshold to trigger the warning style
const warningThreshold = 100;
// Flag to show remaining characters instead of the count
const showRemaining = true;
// Add event listener to update the character count on input
textInput.addEventListener('input', () => {
// Get the current length of the text input
const length = textInput.value.length;
// Update the character count or remaining characters
if (showRemaining) {
charCount.textContent = maxLength - length;
} else {
charCount.textContent = length;
}
// Apply warning style if the threshold is reached
if (length > warningThreshold) {
charCount.classList.add('warning');
} else {
charCount.classList.remove('warning');
}
// Display custom message when the limit is reached
if (length >= maxLength) {
charCount.textContent = "You have reached the limit!";
} else if (length > warningThreshold) {
charCount.textContent = `Warning: Only ${maxLength - length} characters left`;
} else {
charCount.textContent = `Characters: ${length}`;
}
});
Practical Usage
This character counter can be practically used in various applications, such as:
- Social Media Posts: To restrict the length of posts.
- Text Editors: To provide feedback on document length.
- Forms: To limit the input in text fields or areas.
Customizable Options
To make the character counter more flexible and adaptable to different use cases, we can add several customizable options. Here are five options with detailed descriptions:
Name | Description |
---|---|
maxLength | Sets the maximum number of characters allowed in the textarea. This can be specified using the maxlength attribute in HTML or dynamically through JavaScript. If the limit is reached, further typing is prevented. |
counterColor | Allows customization of the text color of the character counter. This can be set via CSS by changing the color property of the .counter class. It can also be dynamically changed using JavaScript based on the character count. |
warningThreshold | Specifies a threshold for the character count that triggers a warning. When the character count exceeds this threshold, the counter’s appearance can change (e.g., color turns red) to alert the user. This can be implemented using JavaScript to add or remove a CSS class. |
showRemaining | Determines whether the counter displays the number of characters typed or the number of remaining characters. This can be toggled by a boolean variable in JavaScript, updating the display accordingly. |
customMessages | Allows setting custom messages for different character count states (e.g., “You have reached the limit”). These messages can be displayed dynamically using JavaScript based on the current character count. |
Implementing Customizable Options
1. maxLength
To set a maximum length for the input, you can use the maxlength
attribute directly in the HTML:
<textarea id="textInput" maxlength="150" placeholder="Type something..."></textarea>
Alternatively, you can dynamically set this attribute in JavaScript if needed:
textInput.setAttribute('maxlength', 150);
2. counterColor
To customize the counter’s color, update the CSS:
.counter {
font-size: 14px;
color: #333; /* Default color */
}
.counter.warning {
color: red; /* Warning color */
}
You can also dynamically change the color using JavaScript based on the character count:
textInput.addEventListener('input', () => {
const length = textInput.value.length;
if (length > warningThreshold) {
charCount.style.color = 'red'; // Warning color
} else {
charCount.style.color = '#333'; // Default color
}
});
3. warningThreshold
Add a warning threshold in JavaScript to change the counter’s appearance:
const warningThreshold = 100;
textInput.addEventListener('input', () => {
const length = textInput.value.length;
charCount.textContent = length;
if (length > warningThreshold) {
charCount.classList.add('warning');
} else {
charCount.classList.remove('warning');
}
});
4. showRemaining
Toggle between showing characters typed and remaining characters:
const maxLength = 150;
const showRemaining = true;
textInput.addEventListener('input', () => {
const length = textInput.value.length;
if (showRemaining) {
charCount.textContent = maxLength - length;
} else {
charCount.textContent = length;
}
});
5. customMessages
Display custom messages based on character count:
const maxLength = 150;
const warningThreshold = 100;
textInput.addEventListener('input', () => {
const length = textInput.value.length;
if (length >= maxLength) {
charCount.textContent = "You have reached the limit!";
} else if (length > warningThreshold) {
charCount.textContent = `Warning: Only ${maxLength - length} characters left`;
} else {
charCount.textContent = `Characters: ${length}`;
}
});
Adding More Customizable Options
6. Dynamic Placeholder Text
To make the placeholder text dynamic based on user interaction, you can update it using JavaScript. For instance, change the placeholder text based on the focus and blur events:
textInput.addEventListener('focus', () => {
textInput.placeholder = "Start typing...";
});
textInput.addEventListener('blur', () => {
textInput.placeholder = "Type something...";
});
7. Character Limit Alert
You can add an alert box to notify users when they reach the maximum character limit. This enhances user experience by providing immediate feedback.
textInput.addEventListener('input', () => {
const length = textInput.value.length;
if (length >= maxLength) {
alert("You have reached the maximum character limit!");
}
});
8. Counter Position
You might want to change the position of the counter based on your layout. For example, place the counter above the textarea:
<div class="counter-container">
<div class="counter">Characters: <span id="charCount">0</span></div>
<textarea id="textInput" maxlength="150" placeholder="Type something..."></textarea>
</div>
Questions and Answers
Q: How can I modify the counter to display remaining characters?
A: You can modify the script to subtract the current character count from a predefined limit, as shown in the showRemaining
option implementation.
Q: Can I style the counter differently for different character counts?
A: Yes, you can use JavaScript to add classes based on the count and style those classes in CSS, as demonstrated in the warningThreshold
option.
Q: How can I make the counter work for multiple text areas?
A: Add unique IDs to each textarea and counter, and use JavaScript to handle each pair separately. You can loop through each textarea and attach event listeners dynamically.
Q: Is it possible to limit the input to a certain number of characters?
A: Yes, you can use the maxlength
attribute in HTML or implement a check in the JavaScript event listener to prevent further input once the limit is reached.
Q: How can I count words instead of characters?
A: Modify the JavaScript to split the input text by spaces and count the resulting array elements. For example:
textInput.addEventListener('input', () => {
const wordCount = textInput.value.split(/\s+/).filter(word => word.length > 0).length;
charCount.textContent = `Words: ${wordCount}`;
});
Related Subjects
1. Form Validation with JavaScript
Form validation ensures that user inputs are accurate and complete before submission. For more information, check this tutorial on form validation.
2. JavaScript Event Handling
Understanding event handling in JavaScript is crucial for interactive web development. Explore more about event handling here.
3. CSS Flexbox
Flexbox is a powerful layout tool in CSS. Learn more about Flexbox here.
4. HTML5 Input Attributes
HTML5 provides several useful attributes for form inputs. Discover more here.
Conclusion
Creating a character counter using JavaScript, HTML, and CSS is straightforward and highly useful for enhancing user experience in text inputs. By following the steps outlined in this blog, you can easily implement this feature in your projects. The customizable options allow you to adapt the counter to different requirements, making it a versatile tool. Additionally, you can expand its functionality with more advanced features, such as dynamic placeholder text, character limit alerts, and flexible counter positioning. Feel free to try the code yourself and customize it according to your needs. If you have any questions, please leave a comment!