Introduction
Countdown timers are essential in various applications, such as event countdowns, sales promotions, and task deadlines. This guide will show you how to create a simple and effective countdown timer using JavaScript, HTML, and CSS. We’ll cover the entire process, from setting up the HTML structure to styling with CSS and adding functionality with JavaScript. Additionally, we’ll discuss several customization options to tailor the timer to your specific needs, including responsive design, different themes, and additional functionalities like alerts and notifications.
Building the Countdown Timer
Step 1: Setting Up HTML
First, we’ll create the basic structure of our countdown timer using HTML. The HTML will include a container for the timer display.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Countdown Timer</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="timer">
<div id="days" class="time-section">
<span class="number">00</span>
<span class="label">Days</span>
</div>
<div id="hours" class="time-section">
<span class="number">00</span>
<span class="label">Hours</span>
</div>
<div id="minutes" class="time-section">
<span class="number">00</span>
<span class="label">Minutes</span>
</div>
<div id="seconds" class="time-section">
<span class="number">00</span>
<span class="label">Seconds</span>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Styling with CSS
Next, we’ll style the countdown timer to make it visually appealing. We’ll use CSS for this purpose.
/* styles.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
#timer {
display: flex;
justify-content: center;
align-items: center;
background: #333;
color: white;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.time-section {
text-align: center;
margin: 0 10px;
}
.number {
font-size: 2em;
display: block;
}
.label {
font-size: 1em;
color: #bbb;
}
Step 3: Adding Functionality with JavaScript
Now, we’ll add the JavaScript code to make the countdown timer functional. This script will calculate the time remaining until a specified date and update the timer display accordingly.
// script.js
// Set the date we're counting down to
const countdownDate = new Date("Dec 31, 2024 23:59:59").getTime();
// Update the count down every 1 second
const x = setInterval(function() {
// Get today's date and time
const now = new Date().getTime();
// Find the distance between now and the countdown date
const distance = countdownDate - now;
// Time calculations for days, hours, minutes, and seconds
const days = Math.floor(distance / (1000 * 60 * 60 * 24));
const hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((distance % (1000 * 60)) / 1000);
// Display the result in the corresponding elements
document.getElementById("days").querySelector('.number').innerText = days;
document.getElementById("hours").querySelector('.number').innerText = hours;
document.getElementById("minutes").querySelector('.number').innerText = minutes;
document.getElementById("seconds").querySelector('.number').innerText = seconds;
// If the countdown is finished, write some text
if (distance < 0) {
clearInterval(x);
document.getElementById("timer").innerHTML = "EXPIRED";
}
}, 1000);
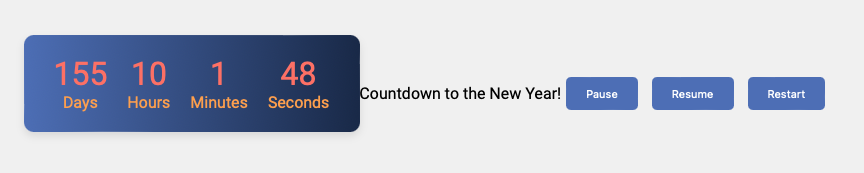
Customization Options
To make your countdown timer unique and suited to your needs, you can customize it in several ways:
Changing the Countdown Date
Description
To change the countdown date, you only need to modify the countdownDate
variable in the JavaScript code. This is helpful if you want the timer to count down to a different event or deadline.
Implementation
In the script.js
file, locate the line where the countdownDate
is set and change it to your desired date and time.
const countdownDate = new Date("Jan 1, 2025 00:00:00").getTime();
Styling the Timer
Description
Customizing the timer’s appearance can make it more visually appealing and aligned with your website’s design. You can modify various CSS properties, such as background color, font size, and spacing.
Implementation
Open the styles.css
file and change the CSS rules. For example, to change the background color and font size:
#timer {
background: #444;
font-size: 1.5em;
}
Adding an Alert Message
Description
An alert message can notify users when the countdown reaches zero. This is useful for drawing attention to the end of the countdown period.
Implementation
Modify the JavaScript to include an alert when the countdown ends.
if (distance < 0) {
clearInterval(x);
document.getElementById("timer").innerHTML = "EXPIRED";
alert("The countdown has ended!");
}
Adding Sound Notification
Description
A sound notification can be an effective way to alert users when the countdown ends, especially if they are not actively looking at the screen.
Implementation
- Add an audio element to your HTML:
<audio id="endSound" src="alert.mp3" preload="auto"></audio>
- Modify the JavaScript to play the sound when the countdown ends:
if (distance < 0) {
clearInterval(x);
document.getElementById("timer").innerHTML = "EXPIRED";
document.getElementById("endSound").play();
}
Responsive Design
Description
Making your countdown timer responsive ensures that it looks good on all devices, including smartphones, tablets, and desktops. This is achieved using CSS media queries.
Implementation
Add CSS media queries to adjust the timer’s layout for smaller screens.
@media (max-width: 600px) {
#timer {
flex-direction: column;
padding: 10px;
}
.time-section {
margin: 5px 0;
}
}
Custom Fonts and Colors
Description
Using custom fonts and colors can enhance the visual appeal of your timer. You can integrate fonts from services like Google Fonts and use CSS to apply them.
Implementation
- Include a Google Font in your HTML:
<link href="https://fonts.googleapis.com/css2?family=Roboto:wght@300;400;700&display=swap" rel="stylesheet">
- Update your CSS to use the new font and colors:
body {
font-family: 'Roboto', sans-serif;
}
#timer {
background: linear-gradient(90deg, #4b6cb7, #182848);
color: #fff;
}
.number {
color: #ff6f61;
}
.label {
color: #ff9f43;
}
Displaying Additional Information
Description
Adding elements such as messages or buttons can provide more context or interactivity to your countdown timer. For example, you can display a message about the event or provide a button to restart the countdown.
Implementation
- Add additional elements to your HTML:
<div id="message">Countdown to the New Year!</div>
<button id="restartButton">Restart</button>
- Update your JavaScript to handle the button click and restart the countdown:
document.getElementById("restartButton").addEventListener("click", function() {
const newCountdownDate = new Date("Dec 31, 2025 23:59:59").getTime();
countdownDate = newCountdownDate;
startCountdown();
});
function startCountdown() {
const x = setInterval(function() {
// existing code to calculate and display the countdown
}, 1000);
}
startCountdown();
Adding Time Zone Support
Description
If your countdown timer needs to be accurate across different time zones, you can adjust it using JavaScript to consider the local time zone of the event.
Implementation
Use the toLocaleString
method in JavaScript to adjust the countdown date for a specific time zone.
const countdownDate = new Date(new Date("Dec 31,
2024 23:59:59").toLocaleString("en-US", {timeZone: "America/New_York"})).getTime();
Adding Pause and Resume Functionality
Description
Providing the ability to pause and resume the countdown timer adds flexibility for users who might need to halt the countdown temporarily.
Implementation
- Add pause and resume buttons to your HTML:
<button id="pauseButton">Pause</button>
<button id="resumeButton">Resume</button>
- Update your JavaScript to handle the pause and resume functionality:
let paused = false;
document.getElementById("pauseButton").addEventListener("click", function() {
paused = true;
});
document.getElementById("resumeButton").addEventListener("click", function() {
paused = false;
});
const x = setInterval(function() {
if (!paused) {
// existing code to calculate and display the countdown
}
}, 1000);
Practical Usage of the Countdown Timer
This countdown timer can be used in various scenarios. For instance, you can embed it on a webpage to count down to a product launch or an event. By changing the countdownDate
in the JavaScript code, you can easily set a different target date and time.
Example
Let’s say you want to create a countdown timer for New Year’s Eve. You would set the countdownDate
to "Dec 31, 2024 23:59:59"
, as shown in the example above. The timer will then display the remaining time until that date.
Questions and Answers
Q: How do I change the countdown date?
A: Change the value of countdownDate
in the JavaScript code to your desired date and time.
Q: Can I style the timer differently?
A: Yes, you can customize the CSS in styles.css
to change the appearance of the timer.
Q: What happens when the countdown reaches zero?
A: The script clears the interval and changes the timer display to “EXPIRED.”
Q: How can I make the timer responsive?
A: Use CSS media queries to adjust the timer’s style for different screen sizes.
Q: Can I use this timer for multiple events?
A: Yes, you can create multiple instances of the timer by duplicating the HTML structure and modifying the JavaScript accordingly.
Q: How do I add a sound notification?
A: Include an audio element in your HTML and use JavaScript to play it when the countdown ends.
Q: Can I add a message when the countdown ends?
A: Yes, you can add a custom message in the JavaScript code to be displayed when the countdown ends.
Q: How do I make the timer display in a different language?
A: Modify the label
elements in the HTML to the desired language.
Q: Can I use a custom font for the timer?
A: Yes, you can include custom fonts using services like Google Fonts and update your CSS accordingly.
Q: How do I restart the countdown without refreshing the page?
A: Add a button to restart the countdown and handle the logic in JavaScript to set a new countdown date and restart the interval.
Q: Can I add pause and resume functionality?
A: Yes, you can add buttons to pause and resume the countdown timer and update the JavaScript accordingly.
Q: How can I adjust the timer for different time zones?
A: Use the toLocaleString
method in JavaScript to adjust the countdown date for specific time zones.
Related Subjects
- JavaScript Date Object: Learn more about the Date object and its methods. MDN Web Docs
- CSS Flexbox: Understand how to use Flexbox for creating flexible and responsive layouts. CSS-Tricks
- JavaScript setInterval() Method: Explore how to use
setInterval()
for running code at specified intervals. W3Schools - HTML Structure: Learn best practices for structuring HTML documents. W3C
- JavaScript Event Listeners: Discover how to use event listeners to handle user interactions. MDN Web Docs
- CSS Media Queries: Learn how to use media queries to create responsive designs. MDN Web Docs
Conclusion
Creating a countdown timer with JavaScript, HTML, and CSS is straightforward and versatile. You can customize the timer to suit different applications and styles, including changing the countdown date, adding alerts and sound notifications, and making the timer responsive. Try implementing this countdown timer in your projects and feel free to ask any questions in the comments.